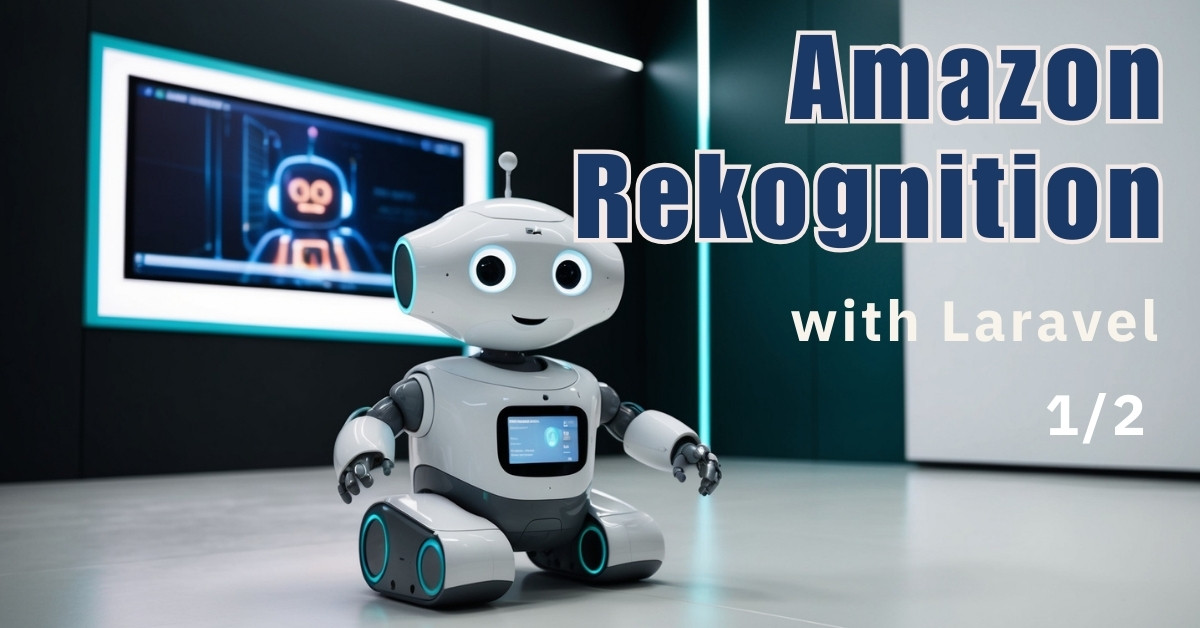
Analyzing videos with Amazon Rekognition and Laravel (Part 1)
Tom Oehlrich
Amazon AWS offers a great way to analyze not only images but also videos. We will use Amazon Rekognition to get a couple of labels that are describing our video as well as the timestamp at which these labels are occurring.
The initial setup and the asynchronous workflow of the recognition workflow can be a little bit tricky at first though.
As always there is more than one way to achieve what we want within the vast amount of AWS services and with PHP.
So this is what we are going to do:
In part 1 (this post) we are setting up Amazon Rekognition, IAM, S3, Simple Notification Service (SNS) and deal with the necessary rights.
In part 2 we are starting a new Laravel project that provides a video file upload to an S3 bucket and starts a label detection analysis with Amazon Rekognition.
We will write a command that checks for the results of the video analysis and that can be executed by a cronjob.
The results will be displayed in a list of uploaded videos and the corresponding detailed info pages.
Note: For the sake of this tutorial, we are using full access rights for the various Amazon services. In a productive environment we would want to restrict rights to what is really necessary.
Note: We are using the same AWS region for everything we are doing on AWS. Otherwise Amazon Rekognition won't be able to communicate with our S3 bucket.
Setting up AWS Services
First let's add a new user in IAM.
- Go to Services -> IAM -> Users
- Click on "Add user"
- Add a name for the user, e.g. "RekognitionUser".
- The Access Type is "Programmatic access".
- Proceed to "Permissions".
- Under "Permissions" click the button "Attach existing policies directly".
- Search for "Amazon Rekognition".
- Tick the checkbox for "AmazonRekognitionFullAccess".
- Next search for "S3".
- Tick the checkbox "AmazonS3FullAccess".
- Proceed to "Review" by skipping the "Tags" page.
- Click on "Create User".
- Now we can download a CSV with the Access key ID and Secret Access Key that we will need later.
Let's switch to Amazon S3 and create a bucket. Other than with image analysis videos processed by Amazon Rekognition have to be stored in S3.
- Go to Services -> S3.
- Click on "Create Bucket".
- Enter a bucket name, e.g. rekbucket4711. The bucket name has to be unique all over AWS.
- Choose an AWS region but make sure to stick to that very region while configuring the Amazon services.
- Proceed to "Permissions" and leave everything as it is.
- Click on "Next" and then "Create Bucket"
Next we will configure the Simple Notification Service (SNS).
SNS or Simple Notification Service is a service that receives the analysis results from the Amazon Rekognition process. The PHP SDK makes it easy to check for the analysis progress and get the results when finished. We will do that using a cronjob.
In a real live application there are a couple of advanced ways of handling this.
We could have SNS push the rekognition results to our own HTTP endpoint and process them there.
We could couple a Simple Queue Service (SQS) with our SNS and then benefit from being able to pull data from SQS.
We could connect AWS Lambda with the SNS and write a Lambda function that deals with the results.
To use SNS we have to create a SNS topic first.
- Go to Services -> SNS.
- Click on "Create topic".
- Enter a topic name, e.g. "RekognitionTopic".
- Click on "Create Topic".
- Write down the Topic ARN in the form of arn:aws:sns:eu-west-1:123456789012:RekognitionTopic for later use.
To grant Amazon Rekognition publishing permissions to the Amazon SNS topic we have to apply an IAM role to it and adjust some permissions.
- Go back to Services -> IAM -> Roles
- Click on "Create role".
- Stay with "AWS service" and select "Rekognition".
- Proceed to "Review" by skipping "Permissions" and "Tags".
- Enter a name for the role, e.g. "RekognitionRole".
- Click on "Create Role".
- Click on the newly created role and write down the role ARN in the form of "arn:aws:iam::123456789012:role/RekognitionRole".
- Go to Services -> IAM -> Users
- Click on the username you are using for this tutorial.
- Click on "Add inline policy".
- Activate the tab "JSON".
- Enter the following JSON snippet in the text area and use your own Rekognition Service Role ARN.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"iam:PassRole"
],
"Resource": "arn:aws:iam::166755042369:role/RekognitionRole"
}
]
}
- Click on "Review policy".
- Enter a name for the policy, e.g. "RekognitionPolicy"
- Click on "Create policy".
That's it.
We should now be ready to proceed with the fun part in Laravel in part 2 of this tutorial.